[Spring Boot] PostgreSQL 연결을 위한 JPA 설정
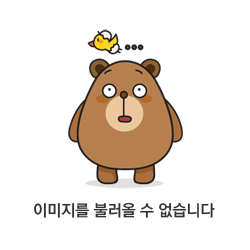
개발환경
- Eclipse IDE 2022-06
- Spring Boot 2.7.2
- Gradle 7.0
- Lombok
- PostgreSQL
기본 Spring Boot 프로젝트를 생성 시 JPA를 추가하였거나 build.gradle 파일에 JPA 추가로 지정한 경우 그에 따른 데이터베이스 설정이 없으면 프로젝트 구동 시 아래와 같은 오류가 발생한다.
Spring Boot에서 JPA를 설정하여 PostgeSQL에 연결하는 방법을 알아보자.
implementation 'org.springframework.boot:spring-boot-starter-data-jpa'
*************************** APPLICATION FAILED TO START *************************** Description: Failed to configure a DataSource: 'url' attribute is not specified and no embedded datasource could be configured. Reason: Failed to determine a suitable driver class Action: Consider the following: If you want an embedded database (H2, HSQL or Derby), please put it on the classpath. If you have database settings to be loaded from a particular profile you may need to activate it (no profiles are currently active).
- Package Explorer
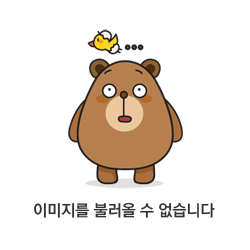
- build.gradle
plugins { id 'org.springframework.boot' version '2.7.2' id 'io.spring.dependency-management' version '1.0.12.RELEASE' id 'java' id 'war' } group = 'com.jpa' version = '0.0.1-SNAPSHOT' sourceCompatibility = '17' repositories { mavenCentral() } dependencies { implementation 'org.springframework.boot:spring-boot-starter-web' implementation 'org.springframework.boot:spring-boot-starter-data-jpa' implementation 'org.postgresql:postgresql' compileOnly 'org.projectlombok:lombok' annotationProcessor 'org.projectlombok:lombok' providedRuntime 'org.springframework.boot:spring-boot-starter-tomcat' testImplementation 'org.springframework.boot:spring-boot-starter-test' } tasks.named('test') { useJUnitPlatform() }
- User.java
package com.example.jpa.persistence.model; import javax.persistence.Column; import javax.persistence.Entity; import javax.persistence.Id; import javax.persistence.Table; import org.hibernate.annotations.DynamicUpdate; import lombok.AccessLevel; import lombok.Getter; import lombok.NoArgsConstructor; import lombok.Setter; @NoArgsConstructor(access = AccessLevel.PROTECTED) @Getter @Entity @Table(name = "tb_user") @DynamicUpdate public class User { @Id @Column(name = "id") private String id; @Setter @Column(name = "password") private String password; @Setter @Column(name = "name") private String name; }
- application.properties
## database spring.datasource.driver-class-name=org.postgresql.Driver spring.datasource.url=jdbc:postgresql://xxx.xxx.xxx.xxx:5432/playground spring.datasource.username=jiurinie spring.datasource.password=password ## jpa spring.jpa.show-sql=true spring.jpa.hibernate.ddl-auto=create spring.jpa.properties.hibernate.format_sql=true spring.jpa.properties.hibernate.jdbc.lob.non_contextual_creation=true ## logging logging.level.org.hibernate.sql=debug logging.level.org.hibernate.type.descriptor.sql.spi=trace
spring.datasource.driver-class-name : 드라이버 클래스 이름
spring.datasource.url=jdbc:postgresql://[ip]:[port]/[DB name]
spring.datasource.username=[db user name]
spring.datasource.password=[db user password]
spring.jpa.show-sql : 실행 SQL쿼리문 Console 출력 여부
spring.jpa.hibernate.ddl-auto (실제 운영서버에서는 create, create-drop, udpate를 설정 하지 않도록 주의한다.)
- none : 아무것도 실행하지 않음 (기본값)
- create : DB Session Factory 연결 시 drop 실행 후 create
- create-drop : DB session factory 연결 시 drop 실행 후 create, DB Session Factory 종료 시 drop
- update : 변경된 스키마 부분만 수정하여 반영
- validate : 변경된 스키마 부분 검증하여 출력, 변경된 부분이 있으면 서버 구동 종료
spring.jpa.properties.hibernate.format_sql : 가독성 향상을 위햔 SQL Formatting
spring.jpa.properties.hibernate.jdbc.lob.non_contextual_creation : PostgreSQL 접속시 아래와 같은 경고 출력 제거
Caused by: java.sql.SQLFeatureNotSupportedException: Method org.postgresql.jdbc.PgConnection.createClob() is not yet implemented
logging.level.org.hibernate.sql : 로깅 모드
logging.level.org.hibernate.type.descriptor.sql.spi : trace로 설정 시 파라미터 출력
구동시 아래 출력과 같이 tb_user 테이블이 drop되었다가 다시 create되며 pgAdmin으로 확인하면 tb_user테이블이 생성되어 있다.
. ____ _ __ _ _ /\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \ ( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \ \\/ ___)| |_)| | | | | || (_| | ) ) ) ) ' |____| .__|_| |_|_| |_\__, | / / / / =========|_|==============|___/=/_/_/_/ :: Spring Boot :: (v2.7.2) 2022-07-28 15:55:54.686 INFO 5848 --- [ main] com.example.jpa.JpaApplication : Starting JpaApplication using Java 17.0.3 on USER with PID 5848 (D:\development\workpsace-tistory\jpa\bin\main started by User in D:\development\workpsace-tistory\jpa) 2022-07-28 15:55:54.688 INFO 5848 --- [ main] com.example.jpa.JpaApplication : No active profile set, falling back to 1 default profile: "default" 2022-07-28 15:55:55.009 INFO 5848 --- [ main] .s.d.r.c.RepositoryConfigurationDelegate : Bootstrapping Spring Data JPA repositories in DEFAULT mode. 2022-07-28 15:55:55.018 INFO 5848 --- [ main] .s.d.r.c.RepositoryConfigurationDelegate : Finished Spring Data repository scanning in 3 ms. Found 0 JPA repository interfaces. 2022-07-28 15:55:55.297 INFO 5848 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat initialized with port(s): 8080 (http) 2022-07-28 15:55:55.304 INFO 5848 --- [ main] o.apache.catalina.core.StandardService : Starting service [Tomcat] 2022-07-28 15:55:55.304 INFO 5848 --- [ main] org.apache.catalina.core.StandardEngine : Starting Servlet engine: [Apache Tomcat/9.0.65] 2022-07-28 15:55:55.369 INFO 5848 --- [ main] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring embedded WebApplicationContext 2022-07-28 15:55:55.370 INFO 5848 --- [ main] w.s.c.ServletWebServerApplicationContext : Root WebApplicationContext: initialization completed in 655 ms 2022-07-28 15:55:55.477 INFO 5848 --- [ main] o.hibernate.jpa.internal.util.LogHelper : HHH000204: Processing PersistenceUnitInfo [name: default] 2022-07-28 15:55:55.506 INFO 5848 --- [ main] org.hibernate.Version : HHH000412: Hibernate ORM core version 5.6.10.Final 2022-07-28 15:55:55.604 INFO 5848 --- [ main] o.hibernate.annotations.common.Version : HCANN000001: Hibernate Commons Annotations {5.1.2.Final} 2022-07-28 15:55:55.661 INFO 5848 --- [ main] com.zaxxer.hikari.HikariDataSource : HikariPool-1 - Starting... 2022-07-28 15:55:55.855 INFO 5848 --- [ main] com.zaxxer.hikari.HikariDataSource : HikariPool-1 - Start completed. 2022-07-28 15:55:55.873 INFO 5848 --- [ main] org.hibernate.dialect.Dialect : HHH000400: Using dialect: org.hibernate.dialect.PostgreSQL10Dialect Hibernate: drop table if exists tb_user cascade Hibernate: create table tb_user ( id varchar(255) not null, name varchar(255), password varchar(255), primary key (id) ) 2022-07-28 15:55:56.212 INFO 5848 --- [ main] o.h.e.t.j.p.i.JtaPlatformInitiator : HHH000490: Using JtaPlatform implementation: [org.hibernate.engine.transaction.jta.platform.internal.NoJtaPlatform] 2022-07-28 15:55:56.217 INFO 5848 --- [ main] j.LocalContainerEntityManagerFactoryBean : Initialized JPA EntityManagerFactory for persistence unit 'default' 2022-07-28 15:55:56.240 WARN 5848 --- [ main] JpaBaseConfiguration$JpaWebConfiguration : spring.jpa.open-in-view is enabled by default. Therefore, database queries may be performed during view rendering. Explicitly configure spring.jpa.open-in-view to disable this warning 2022-07-28 15:55:56.429 INFO 5848 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port(s): 8080 (http) with context path '' 2022-07-28 15:55:56.436 INFO 5848 --- [ main] com.example.jpa.JpaApplication : Started JpaApplication in 1.974 seconds (JVM running for 2.436)
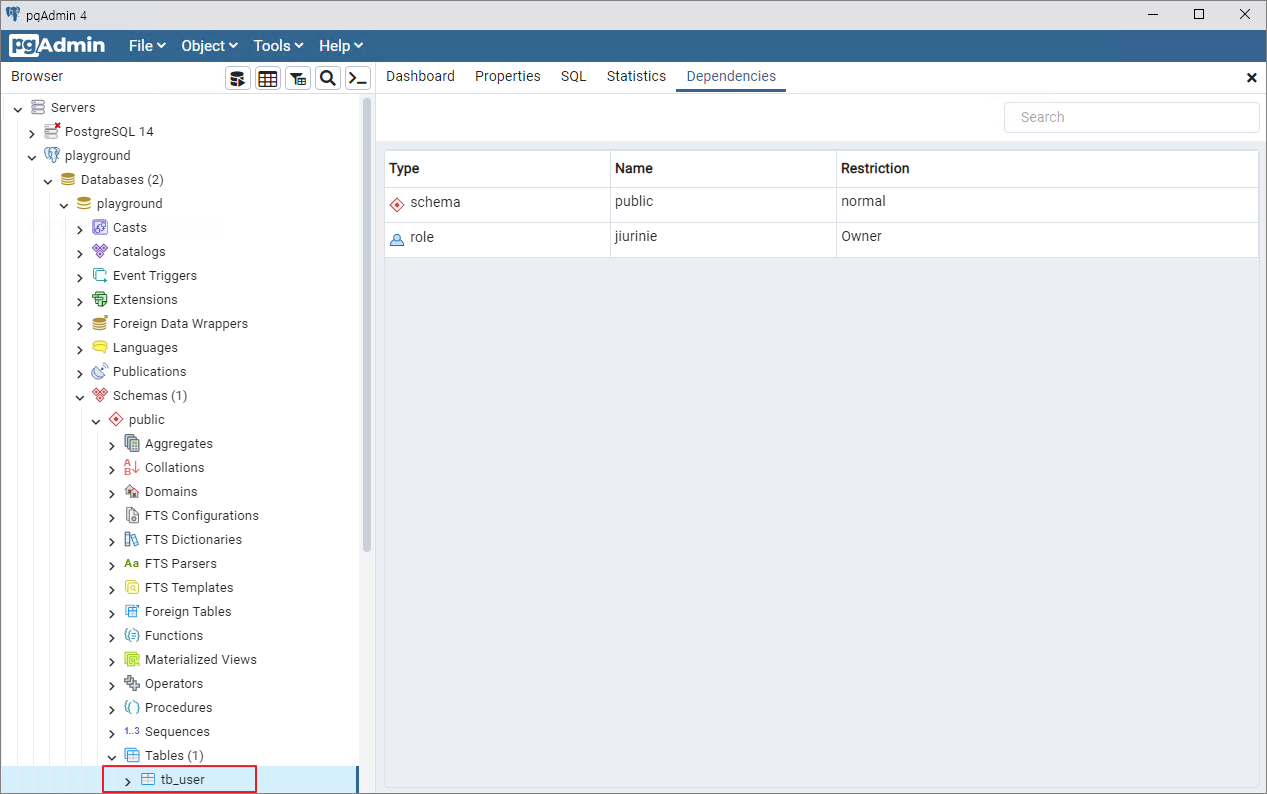
PostgreSQL서버에 접근이 불가능하다면 ?
Windows PostgreSQL서버 외부에서 접속 허용하기
Windows PostgreSQL서버 외부에서 접속 허용하기 PostgreSQL서버는 기본적으로 로컬호스트에서는 설정파일의 변경 없이 접근이 가능하다. 그러나 외부에서의 접근은 허용하지 않는데 외부에서 접근을
jiurinie.tistory.com
pgAdmin4로 PostgreSQL서버 접속하기
pgAdmin4로 PostgreSQL서버 접속하기 Windows에 PostgreSQL14 설치하기 Windows에 PostgreSQL14 설치하기 개발환경을 구축하기 위해 Windows에 PostgreSQL을 설치하려고 한다. 공식 사이트에 접속하여 PostgreSQL..
jiurinie.tistory.com
'Programming > Spring Boot' 카테고리의 다른 글
[Spring Boot] Gradle을 이용한 Profile(개발/운영) 구분 설정 (0) | 2022.12.02 |
---|---|
[Spring Boot] Jasypt를 이용한 암호화 (0) | 2022.11.30 |
Spring Boot 기본 설정 Port, ContextPath, Session Timeout (0) | 2022.07.22 |
Spring Boot 웹 프로젝트 생성 (0) | 2022.07.08 |
Spring Boot urlrewritefilter를 이용한 URL 리다이렉션 (0) | 2022.07.05 |
댓글